Build Incredibly Powerful REST APIs with HyperMap
HyperMap is a new format for REST APIs that lets you execute JavaScript and WebAssembly on the client. This gives you access to modern Web APIs like WebSockets
or fetch
to build APIs that can dynamically update with asynchronous or streaming data, or even push whole computations closer to the data.
Who should use it?
Anyone building REST APIs can benefit from moving to HyperMap. The integration path is really straightforward and your APIs will be much more powerful. If you already use a hypermedia format like HAL or Siren you'll find HyperMap very familiar, with the added benefit of being able to execute code on the client.
HyperMap doesn't yet offer a built-in query capability like GraphQL, but being able execute code on the client can bring some of the benefits that people look for in GraphQL without having to rearchitect your whole server stack.
How does it work?
On the server side, just change your content type to
application/vnd.hypermap+json
and you're set! From there, you can use the
special #
key to add attributes to your API repsonses.
There's a universal client, Mech, which can be used to call any HyperMap API. Instead of writing or generating custom clients for each API, just use Mech. Mech supports sandboxed execution of multiple APIs and has a reactive interface, letting you listen to and respond to API changes as they happen.
Read a guide to migrating to HyperMap in the docs.
What tools does it come with?
There's a Visual Studio Code extension, APM Explorer that lets you navigate HyperMap APIs, and scaffold integration code from a series of interations.
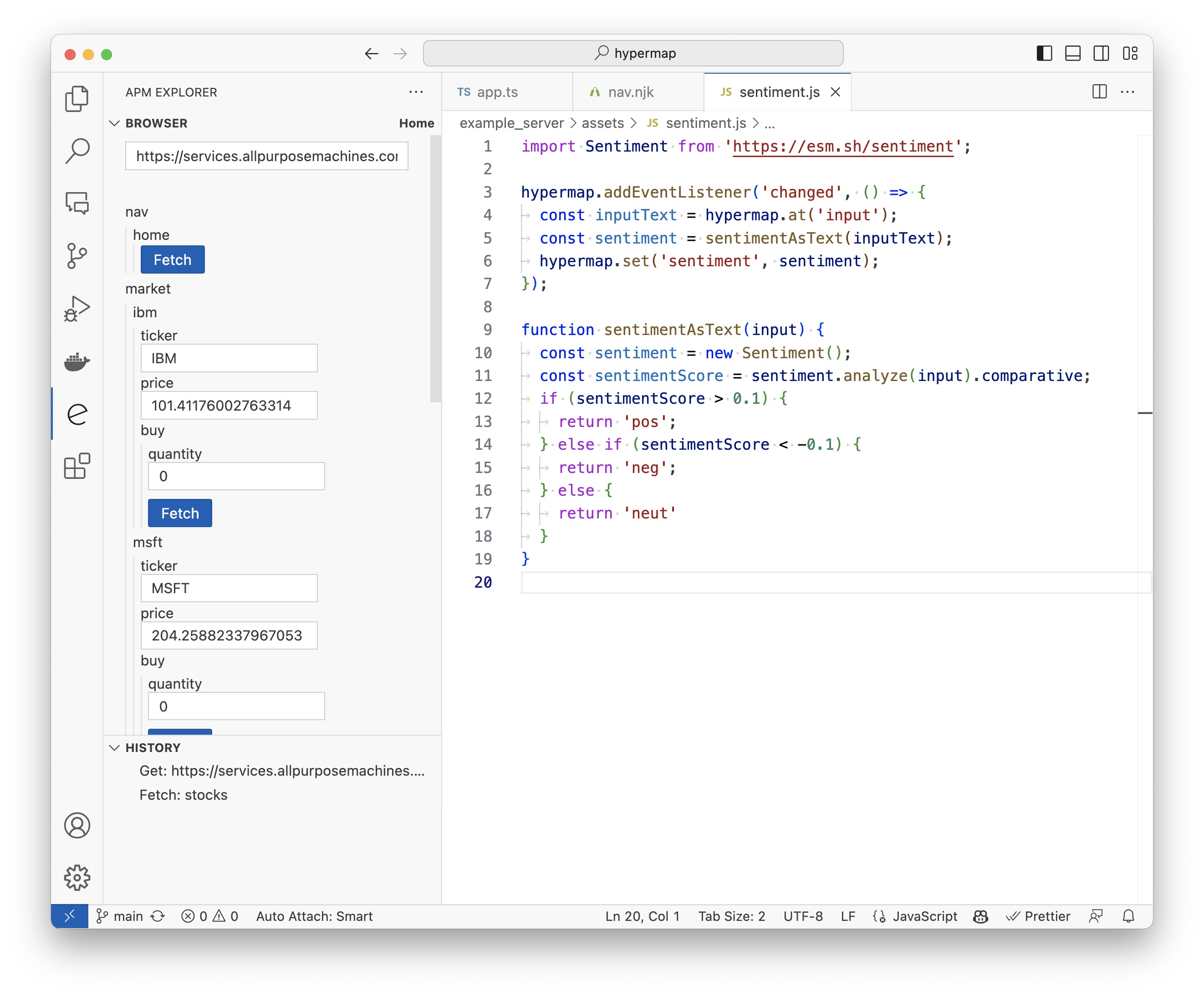
What can I use it for?
Eliminate Webhooks
Webhooks are great for notifying a client that a long running process has completed but assumes you have a publicly reachable URL to POST back to. This isn't the case if you're developing native apps, developing locally, or running on CI.
Instead, with HyperMap you can just create an EventSource and listen to SSEs:
// GET /tickets/2277/
{
"#": {
"script": "/eventHandler.js"
},
"ticketId": "2277",
"status": "pending"
}
// GET /eventHandler.js
const id = hypermap.at('ticketId'); // Fetch HyperMap values
const evtSource = new EventSource(`/tickets/${id}/events`);
evtSource.onmessage = (event) => {
hypermap.set('status', event.data); // Set HyperMap values
};
Apps looking to react to this change can just use an event handler through Mech:
// client.js
const ticketTab = Mech.open('https://example.com/tickets/2277/');
// Mech raises an event whenever the HyperMap changes
ticketTab.on('changed', () => {
console.log(`New status: ${ticketTab.at('status')}`);
});
Unify REST and streaming endpoints
Something that's increasingly common is to ship two APIs: a regular REST one for structured data and a WebSocket one for streaming data. With HyperMap you can have the best of both worlds:
// GET /stocks/
{
"#": {
"script": "/streamHandler.js"
},
"aapl": 180.67,
"meta": 321.91,
"googl": 138.80
}
// GET /streamHandler.js
const stockSocket = new WebSocket('wss://example.com/stocks/');
stockSocket.onmessage = (event) => {
const msg = JSON.parse(event.data);
hypermap.set(msg.ticker, msg.price);
};
Client-side pre-processing
If you need to process data that's private or sensitive you might need to
hard-code some logic in your wrapper/SDK to scrub that information before
sending to your service. With HyperMap you can ship the logic to the client
alongside the API reponse, ensuring it works in any supported language and is
always up to date. See the privacy-preserving text sentiment analysis demo in
the example_server
.
Is commerical support available?
Yes! Just email us to learn more.